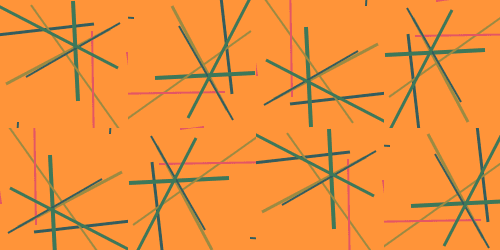
Server Sent Events (SSE)
What is it?
Server-Sent Events (SSE) enables real-time communication between the server and client. It allows the server to send updates directly to the client without constant requests, eliminating frequent polling and reducing network traffic.
One major advantage of SSE is its simplicity. Unlike more complex solutions like WebSockets, SSE requires minimal setup and works over standard HTTP connections. This makes it easier to implement and maintain. It is particularly useful for applications that require real-time updates, such as social media feeds and stock market tickers.
Connection
To initiate an SSE connection, the client sends a standard HTTP request to the server. This request includes the necessary headers, such as the Accept header set to text/event-stream. This informs the server that the client is requesting an SSE response.
// Example with JavaScript
const eventSource = new EventSource('http://localhost:3000/sse');
Upon receiving the client's request, the server acknowledges the SSE connection by responding with the appropriate headers:
Content-Type: text/event-stream
Cache-Control: no-cache
Connection: keep-alive
The server usually includes additional event-specific headers such as Event-Id
orcustom headers to provide more context or metadata.
Pushing events
The server formats the event according to the SSE specification. Each event is a plain-text message and follows a specific structure. It starts with the "data" field, which contains the actual content of the event.
// Example with NodeJS
res.write(`data: ${JSON.stringify({ message: "Hello!" })}\n\n`);
Handling events
As the server sends the event, the client's browser receives it and triggers the associated event listener or callback function. The server can continue pushing events to the client as long as the SSE connection remains open and decides when and how often to send events based on the application's logic and the availability of new data.
// Handling events (client-side)
eventSource.addEventListener('message', (event) => {
const eventData = JSON.parse(event.data);
console.log('Event received:', eventData);
});
SSE vs. WebSockets
SSE is based on HTTP, which means that each event sent from the server to the client is wrapped in an HTTP response. The delay introduced in SSE is primarily related to the request-response cycle.
WebSockets use a more efficient, lower-overhead protocol that allows for full-duplex, bidirectional communication. This direct, persistent connection enables real-time data exchange with minimal latency.
It's important to consider the specific requirements of your application and evaluate the technologies to determine which one better suits your case.
You can find the full code here.