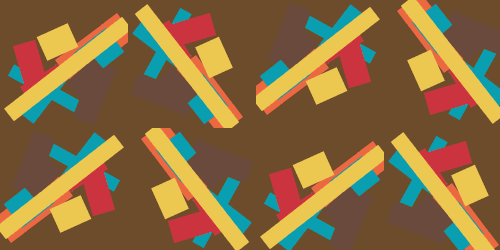
Elegant objects vs. Data Containers
After several years working as backend (and mobile) programmer mostly in the Java Virtual Machine ecosystem, I have realized that no one of those procedural MVC/MVP/MVVM patterns have made me feel confortable when implementing new features or big changes in a project.
Also, ER-ending classes (Controller, Manager, Helper…), that are well accepted and used in many frameworks, don’t help with that either: they will get bigger and bigger and you will have to segregate them without any logical criteria. And when that happens, you’re screwed. Maintainability becomes really hard.
Last months, I have been heavily influenced by Yegor Bugayenko and what he claims: “pure” object oriented programming. Instead of treating objects as simple (and silly) data containers/data structures, we should give them the power and trust them. Convert them in elegant objects.
Can you see any difference between this Java code:
public class User {
private String id;
private String username;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
and this C code:
struct User{
char id[50];
char username[50];
};
Not at all. Because there is not. However, we write objects like above and are convinced we are object oriented developers. But we aren’t. We must think of objects as something more than simple in-memory data structures exposing its state to everybody. Objects should wrap its real-life representation. Keep reading.
Let’s say we need to write a class File (in Java) with a method content() that returns its content in a byte array. A first implementation could be something like this:
public class File {
private String path;
private byte[] content;
public File(String path, byte[] content) {
this.path = path;
this.content = content;
}
//getters and setters
}
public class FileSystem {
public byte[] getFileContent(String path) {
return /* read file bytes from disk */;
}
And we would use it this way:
FileSystem fs = new FileSystem();
String path = "/tmp/conf.xml";
File confFile = new File(path, fs.getFileContent(path));
Done. To be honest, I think this implementation is a mess. Don’t you? If we change the content of file /tmp/conf.xml, configFile object becomes inconsistent. File class is not representing a real file, but a bunch of bytes. It has been reduced to a data container. A C struct. It can’t be considered an object at all. This is a nice implementation for the same class:
public interface File {
byte[] content();
}
public final class FileSystemFile implements File {
private final String path;
public FileSystemFile(String path) {
this.path = path;
}
@Override
public byte[] content() {
return /_ read file bytes from disk _/;
}
}
And we use it this way:
```java
File confFile = new FileSystemFile("/tmp/conf.xml");
Much better, right? Now, configFile is an elegant object. We have converted File type in an interface. This way, we can have more implementations like RemoteFile, if needed.
You may think that this implementation is not efficient because each time we call content() method, a disk read is performed. It may be slow depending on the system and the application requirements. You are right.
So, now it’s when object composition and decorator design pattern come into action.
We are going to create a class CachedFile that wraps a File and cache its content, so just one disk read will be performed:
public final class CachedFile implements File {
private final File origin;
private byte[] cached;
public CachedFile(File origin) {
this.origin = origin; this.cached = null;
}
@Override public byte[] content() {
if (this.cached == null) {
this.cached = origin.content(); //real disk read
}
return this.cached;
}
}
(This is a very naive cache implementation. Also, you must never use null. I did it just for the example.)
And we use it this way:
File confFile = new CachedFile(new FileSystemFile("/tmp/conf.xml"));
It looks nice now. What if I tell you that this approach can be applied to database objects too?
Let’s back to the 'User' example. A real, stored in database, User would look like this:
public interface User {
public String id();
public String username();
}
public DatabaseUser implements User {
private final String id;
private final Database db;
public DatabaseUser(String id) {
this.id = id;
this.db = db;
}
@Override
public String id() {
return this.id;
}
@Override
public String username() {
return /* SELECT username FROM users WHERE id = this.id */;
}
}
Now, a User
is not just a bunch of data. Its state is not being exposed. It is an elegant object. Again, we can "decorate" it with a cache or whatever we want to throughput optimization.
If you liked it this approach of OOP, I strongly recommend you to read Yegor’s posts and his book Elegant Objects.