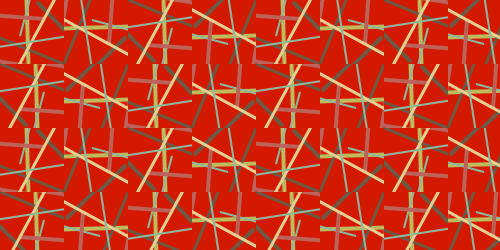
Microservice Pattern: API Gateway
Microservice architectures are here to stay and, when implement them, we need to face some troubles we don’t suffer in monolithic systems. How to fetch and aggregate data from several services to serve a single request is one of them, and API Gateway pattern is what we need to solve it.
First, we have a Order Microservice, where, via HTTP call, we can get information from an order:
GET http://order_service/orders/1
{
id: 1,
description: "This is the order’s description",
customerId: 56,
lines: [
{ description: "item 1", price: 3.5 },
{ description: "item 2", price: 2 }
],
total: 5.5
}
Order Microservice only works with orders, not with customers; that’s why the order doesn’t know anything about the customer but her ID. Then, we have a Customer Microservice, where we can get complete information about that customer:
GET http://customer_service/customers/24
{
id: 24,
name: "John Doe",
email: "john@protonmail.com"
}
Now, we want to expose our services as a public API so our applications, or external ones, can consume it. When one client requests information about an order, we want to embed customer information in the response, instead of force him to make another extra request. This data aggregation logic must be made in the API Gateway layer and the response would be something like this:
GET http://api_gateway/orders/1
{
"id": "1",
"description": "This is the order's description",
"lines": [
{
"description": "item 1",
"price": 3.5
},
{
"description": "item 2",
"price": 2
}
],
"customer": {
"id": "56",
"name": "John Doe",
"email": "john@protonmail.com"
}
}
As you see, response constains full data from the customer. API consumer doesn’t know that internally two requests were needed to build the response; it’s transparent to her, and that’s the core idea of API Gateway.
About API Gateway
- It acts as router
- It is the only entry point to our collection of microservices. This way, microservices are not needed to be public, anymore, but are behind an internal network and API Gateway is the responsible of making requests against a service or another one (Service Discovery)
- It acts as a data aggregator: API Gateway fetches data from several services and aggregate it to return a single rich response. Depending on the API consumer, data representation may change according the needs, and here is where Backend For Frontend (BFF) comes into play.
- It is a protocol abstraction layer: API Gateway can be exposed as a REST API or GraphQL or whatever, no matter what protocol or technology is being used internally to communicate with the microservices.
- Error management is centralized: When a service is not available, is getting too slow or something like that, API Gateway can provide data from cache, default responses or make smart decisions to avoid bottlenecks or fatal errors propagation. This keeps the circuit closed (circuit breaker pattern) and makes the system more resilient and reliable.
I have built a naive implementation of an API Gateway, based on the example above, using NodeJS, Express and RxJS. You can find it in this repo.